We will see how we can also show the images for the combo items bases on some conditions.
X12 EDI Examples
ZK Border Layout–Another example
This examples show how you can use ZK Border Layout to show the product information on clicking image.
MVVM–List Item–Hibernate–MySQL–Part 3
Note: This is continuation of my previous article Part 2. Please click here to go to Part 2
In this Part 3, we will enhance our Listing and model window as follows
1. We will include one more column as last named as Actions. This action will contain image such as edit, activate and delete.
2. We will also give hyperlink for the first column. If the user clicks the first column, then we will show the information in the read-only and if the user clicks the edit image in the action column, then we will show the information in the read only and will allow the user to edit and save.
C Workbook
In the year 1999-2002, I was working as faculty in a computer center. During that period, I developed a small VB Application to explain the concept of C. Here are the some screenshots from that VB Project
MVVM Modal window–Pass Parameter and Return values
In this post, we will see how we can pass some values to the modal window when calling from the parent window and also vice versa (i.e) return some values from the modal window to parent window
Project Name : MVVMModalWindow
Project Structure:
Demo.zul
<?page title="new page title" contentType="text/html;charset=UTF-8"?>
<zk>
<window title="MVVM Modal window Passing arguments and retur values"
border="normal" apply="org.zkoss.bind.BindComposer"
viewModel="@id('e') @init('com.demo.UI.demoVM')">
Type any value and Press the Model Window Button
<separator />
Value 1 :
<textbox value="@bind(e.value1)" />
Value 2 :
<textbox value="@bind(e.value2)" />
<button label="Model Window" onClick="@command('showModelWin')" />
</window>
</zk>
package com.demo.UI;
import java.util.HashMap;
import org.zkoss.bind.annotation.BindingParam;
import org.zkoss.bind.annotation.Command;
import org.zkoss.bind.annotation.GlobalCommand;
import org.zkoss.zk.ui.Executions;
import org.zkoss.bind.annotation.NotifyChange;
public class demoVM {
private String value1;
private String value2;
public String getValue1() {
return value1;
}
public void setValue1(String value1) {
this.value1 = value1;
}
public String getValue2() {
return value2;
}
public void setValue2(String value2) {
this.value2 = value2;
}
@Command
public void showModelWin()
{
final HashMap<String, Object> map = new HashMap<String, Object>();
map.put("value1", this.value1 );
map.put("value2", this.value2);
Executions.createComponents("ModelWindow.zul", null, map);
}
@GlobalCommand
@NotifyChange({"value1","value2"})
public void refreshvalues(@BindingParam("returnvalue1") String str1, @BindingParam("returnvalue2") String str2)
{
this.value1 = str1;
this.value2 = str2;
}
}
<?page title="new page title" contentType="text/html;charset=UTF-8"?>
<zk>
<window id="modalDialog"
title="MVVM Modal window Passing arguments and retur values"
width="420px" height="auto" border="normal" minimizable="false"
mode="modal" maximizable="false" closable="true"
action="hide: slideUp" apply="org.zkoss.bind.BindComposer"
onCancel="@command('closeThis')"
viewModel="@id('e') @init('com.demo.UI.ModelWindowVM')">
Change the values and Press the Ok Button to return changed
values.
<separator />
Value 1 :
<textbox value="@bind(e.value1)" />
Value 2 :
<textbox value="@bind(e.value2)" />
<button label="Ok" onClick="@command('save')" />
</window>
</zk>
package com.demo.UI;
import org.zkoss.bind.annotation.Command;
import org.zkoss.bind.annotation.ContextParam;
import org.zkoss.bind.annotation.ContextType;
import org.zkoss.bind.annotation.ExecutionArgParam;
import org.zkoss.bind.annotation.Init;
import org.zkoss.zk.ui.Component;
import org.zkoss.zk.ui.select.Selectors;
import org.zkoss.zk.ui.select.annotation.Wire;
import org.zkoss.zul.Window;
import java.util.HashMap;
import org.zkoss.bind.BindUtils;
import java.util.Map;
public class ModelWindowVM {
@Wire("#modalDialog")
private Window win;
private String value1;
private String value2;
public String getValue1() {
return value1;
}
public void setValue1(String value1) {
this.value1 = value1;
}
public String getValue2() {
return value2;
}
public void setValue2(String value2) {
this.value2 = value2;
}
@Init
public void init(@ContextParam(ContextType.VIEW) Component view,
@ExecutionArgParam("value1") String v1,
@ExecutionArgParam("value2") String v2) {
Selectors.wireComponents(view, this, false);
this.value1 = v1;
this.value2 = v2;
}
@SuppressWarnings({ "unchecked", "rawtypes" })
@Command
public void save() {
Map args = new HashMap();
args.put("returnvalue1", this.value1);
args.put("returnvalue2", this.value2);
BindUtils.postGlobalCommand(null, null, "refreshvalues", args);
win.detach();
}
@Command
public void closeThis() {
win.detach();
}
}
Now you can run the demo.zul file
C++ Workbook
ZK Border Layout–Menu on the left and content on the right.
In this post, let us see how we can load all our project menu in the left and on click of each of menu, then we will load individual zul file on the right side. Here, we are going to use border layout, where north border going to content menu and west border will be used to load the individual zul file.
Many thanks Stephan who helped me to complete this task. Here is the forum thread
http://www.zkoss.org/forum/listComment/18840-Menu-Links-on-Left-Side-and-Page-Content-on-Right-Side?lang=en
MVVM–List Item–Hibernate–MySQL–Part 2
In this post, we will see how we can do the following stuffs
1. Add new person by calling a model window in MVVM and update in the DB
2. On Double click of the records in the list item, edit the existing record by calling a modal window in MVVM and update in the DB
3. Then after edit and add, we will refresh the list. Note, after edit, we no need to do anything, because data binding will take care. But after adding new person, we will refresh the list using Global command

Practice management System– PMS–Billing
Last year I was involved in developing an independent system for PMS using VB6 with sql server 2005. I really enjoyed myself and love to work/complete that project. One of my best period in my life. But unfortunately, we could not able to market the product because of VB6 no longer used widely in the industry.
Here are some of the screens which I like most in my development of VB6
Phone Box–Using j Query and Extending ZK Textbox
Summary
In this post, we will see how we can use jquery with ZK for US Phone number format. And also later on, we will see how we can this as a reusable component by extending the ZK Textbox
Thanks to the following ZK Forum and all the contributors who helped me to create this articlehttp://www.zkoss.org/forum/listComment/17490-How-to-apply-a-mask-in-a-Textbox-not-rendered-by-a-zul-file
MVC Two combo Box – Fill second combo based on first combo selection
We will see how to fill the second combo box based on first combo box selection using MVC Data binding.
MVVM Two combo Box – Fill second combo based on first combo selection
We will see how to fill the second combo box based on first combo box selection using MVVM Data binding.
MVVM–List Item–Hibernate–MySQL–Part 1
In every application, we will always have the listing page where we can list the records from the DB, from there another model window will be used to Add/Edit records.
In this post, we will see how we can do the following stuffs
1. Bind the List Item Using New zk 6 Data binding with MVVM Pattern
2. Retrieve the values from the DB – Mysql using Hibernate as ORM Tool.
3. How to change the color of the particular row based on some condition.
4. How to handle double click event on the List item.
5. How to sort the values in the List Item.
MVC Combo Box Data binding
List Item Double click event on selected Item using MVVM
MVVM Example for Form Validation
List Item on Select, show more details by using selectedItem
List item Change background color for some particular items based on some conditions
List Item Double click event on selected Item
ViewModel Class Java Annotation @Init, @NotifyChange, @Command
In following sections we'll list all syntaxes that can be used in implementing a ViewModel and applying ZK bind annotation.
ZK MVVM CRUD - Part 3
Previous Part 2
In Part 2, we slightly change the retrieve of objects by adding constructor. Now we will move forward. Let us take a look on the output as follows
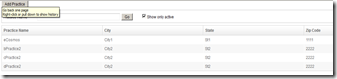
Apart from the listing the records, we also have two search parameters such as Practice name and Show only active or inactive practices. So what we are going to do with this ? Well, if the list is big, then user has to move to particular page to locate the desired practice, instead of that we will filter the practice as soon as user starts typing the first few characters of the practice name. And also, by default we will show all the active (soft delete) records. If the user want to see all the records including deactivated, then on click of check box, we will also do that.
So here are the conditions
Case 1:
If no character present in the Practice name filter text area and also Show only active is unchecked, then let us show all the records from the DB.
Case 2:
If some characters are typed in the practice name filter and check box is unchecked, then show all active and inactive records for the practice whose first characters are matched with the filter text
Case 3:
If no character in practice name filter text area and check box is checked, then we will show all the active practices only.
Case 4:
If both the search parameters contains values, then we will retrieve the records accordingly.
And also, we are going to Apply new ZK Bind Annotation such as @NotifyChange and @Command. Please refer here for examples in this subject.
And also very important, we are not going hit the database for each character is typed or check box is checked or unchecked. First time we will hit the DB to bring the records and then we will do all the filter locally.
Step 1:
From the View, we need to pass the value of Practice name text area and Check box state (checked or unchecked) to the View Model to filter the record. So we will write the following getter and setter method.
private String practiceStartWith;
private boolean showOnlyActive;
public String getPracticeStartWith() {
return practiceStartWith;
}
public void setPracticeStartWith(String practiceStartWith) {
this.practiceStartWith = practiceStartWith;
}
public boolean isShowOnlyActive() {
return showOnlyActive;
}
public void setShowOnlyActive(boolean showOnlyActive) {
this.showOnlyActive = showOnlyActive;
}
Step 2:
Here is the modified PracticeVM.Javapackage UIVMs; import domain.Practice; import java.util.ArrayList; import java.util.List; import org.zkoss.bind.annotation.Command; import org.zkoss.bind.annotation.NotifyChange; import DomainDAO.practiceDAO; public class PracticeListVM { private List<Practice> AllPracticeInDB = null; private List<Practice> filteredPractices = null; private String practiceStartWith; private boolean showOnlyActive = true; public String getPracticeStartWith() { return practiceStartWith; } public void setPracticeStartWith(String practiceStartWith) { this.practiceStartWith = practiceStartWith; } public boolean isShowOnlyActive() { return showOnlyActive; } public void setShowOnlyActive(boolean pActive) { this.showOnlyActive = pActive; } public List<Practice> getprlist() { if (AllPracticeInDB == null) { filteredPractices = new ArrayList<Practice>(); AllPracticeInDB = new practiceDAO().getListingItems(); setShowOnlyActive(true); for (Practice item : AllPracticeInDB) { if (item.getIsActive() == 1) filteredPractices.add(item); } } return filteredPractices; } @NotifyChange("prlist") @Command public void doFilter() { filteredPractices.clear(); /* if no filter characters and check box is un checked. Then get all */ /* 0 and 0 */ if ((practiceStartWith == null || "".equals(practiceStartWith)) && (showOnlyActive == false)) { filteredPractices.addAll(AllPracticeInDB); } /* * if there are filter characters and check box is un checked. Then get * filtered by filter text */ /* 1 and 0 */ if ((practiceStartWith != null && !practiceStartWith.equals("")) && (showOnlyActive == false)) { for (Practice item : AllPracticeInDB) { if (item.getPracticeName().toLowerCase() .indexOf(practiceStartWith.toLowerCase()) == 0) filteredPractices.add(item); } } /* * if no filter characters and check box is checked. Then get only * active practices */ /* 0 and 1 */ if ((practiceStartWith == null || "".equals(practiceStartWith)) && (showOnlyActive == true)) { for (Practice item : AllPracticeInDB) { if (item.getIsActive() == 1) filteredPractices.add(item); } } /* * Both Contains values i.e filter contains some text and check box is * checked */ /* 1 and 1 */ if ((practiceStartWith != null && !practiceStartWith.equals("")) && (showOnlyActive == true)) { for (Practice item : AllPracticeInDB) { if (item.getPracticeName().toLowerCase() .indexOf(practiceStartWith.toLowerCase()) == 0) if (item.getIsActive() == 1) filteredPractices.add(item); } } } }
Step 3:
Here is the modified practiceList.zul
<?page title="Practice List" contentType="text/html;charset=UTF-8"?> <zk> <window apply="org.zkoss.bind.BindComposer" viewModel="@id('vm') @init('UIVMs.PracticeListVM')"> <div> <button label="Add Practice" /> </div> <separator /> <groupbox height="40px"> <label value="Practice Name" /> <space /> <space /> <textbox width="250px" value="@bind(vm.practiceStartWith)" onChange="@command('doFilter')" instant="true" /> <button id="gobutton" label="Go" /> <space spacing="20px" /> <checkbox id="activechk" label="Show only active" checked="@bind(vm.showOnlyActive)" onCheck="@command('doFilter')" /> <space spacing="20px" /> </groupbox> <separator /> <listbox id="PracticeList" model="@load(vm.prlist)"> <listhead sizable="true"> <listheader label="Practice Name" sort="auto" /> <listheader label="City" sort="auto" /> <listheader label="State" sort="auto" /> <listheader label="Zip Code" sort="auto" /> </listhead> <template name="model" var="p1"> <listitem> <listcell label="@load(p1.practiceName)" /> <listcell label="@load(p1.city)" /> <listcell label="@load(p1.state)" /> <listcell label="@load(p1.zipCode)" /> </listitem> </template> </listbox> </window> </zk>
Step 4:
Now we can run and play around both practice name filter and check box.
The following is the execution flow
1. An Instance will be created for our PracticeListVM
2. Since we declared true value initially for showonlyactive, and also we bind our checkbox with showactiveonly, so first set method of showonlyactive will take place.
3. Then our getprList will take place because we bind our list to this get method.
ZK MVVM CRUD - Part 2
Previous Part 1
In the part 1, we have listed all the records from the DB.
I would like to do some changes in the Part 1 without adding any other stuff. Open PracticeDAO. Java and let us have a close look on the following line;
Query q1 = session.createQuery("from Practice ");
Here, we did not specify any columns, so it will fill up the values for all the columns matching with our java bean. But in the list, we need only to display four columns such as Practice Name, city, State and Zip Code.
So how we can retrieve only selected columns in the create query ? You can see this example to achieve the result.
So as per that example, first let us constructors in our practice.java as shown here.
package domain; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.Id; import javax.persistence.Table; @Entity @Table(name = "practice") public class Practice { private Integer practiceID; private String practiceName; private String city; private String ZipCode; private String state; private Integer isActive; // Our default Constructor public Practice() { } // constructor with fields needed for our listing public Practice(Integer pID, String prname, String pCity, String pState, String pZipCode, Integer pIsActive ) { this.practiceID = pID; this.practiceName = prname; this.city = pCity; this.state = pState; this.ZipCode = pZipCode; this.isActive = pIsActive; } @Id @GeneratedValue @Column(name = "PRACTICE_ID") public Integer getPracticeID() { return practiceID; } public void setPracticeID(Integer practiceID) { this.practiceID = practiceID; } @Column(name = "PRACTICE_NAME") public String getPracticeName() { return practiceName; } public void setPracticeName(String practiceName) { this.practiceName = practiceName; } @Column(name = "CITY") public String getCity() { return city; } public void setCity(String city) { this.city = city; } @Column(name = "POSTAL_CODE") public String getZipCode() { return ZipCode; } public void setZipCode(String zipCode) { ZipCode = zipCode; } @Column(name = "STATE_PROVINCE_GEO") public String getState() { return state; } public void setState(String state) { this.state = state; } @Column(name = "IS_ACTIVE") public Integer getIsActive() { if (isActive== null) return 1; else return isActive; } public void setIsActive(Integer isActive) { this.isActive = isActive; } }
Now let us change our DAO as follows
Query q1 = session.createQuery("Select new Practice(practiceID, practiceName,city,state,zipCode,isActive) from Practice ");
Next Part 3
Select only few columns in Hibernate create query API
Step : 1
Set up Java + Hibernate Environment as by this post.
Step : 2
Alter our patient table as shown
Step : 3
Now let go back to our patient.java and add the columns as follows
package mypack;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.Table;
import javax.persistence.Column;
import javax.persistence.Transient;
@Entity
@Table(name = "patient")
public class patient {
private Integer id;
private String firstName;
private String lastName;
private String password;
private String email;
private String address1;
private String address2;
private String city;
private String state;
private String zipCode;
@Transient
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
@Id
@GeneratedValue
@Column(name = "ID")
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
@Column(name = "firstname")
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
@Column(name = "lastname")
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getAddress1() {
return address1;
}
public void setAddress1(String address1) {
this.address1 = address1;
}
public String getAddress2() {
return address2;
}
public void setAddress2(String address2) {
this.address2 = address2;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
public String getZipCode() {
return zipCode;
}
public void setZipCode(String zipCode) {
this.zipCode = zipCode;
}
}
Step : 4
Modify our Test.java file as follows
package mypack;
import java.util.List;
import org.hibernate.Query;
import org.hibernate.Session;
import Utility.HibernateUtil;
public class Test {
public static void main(String[] args) {
getAllRecords();
}
public static void getAllRecords() {
List<patient> allpatients;
Session session = HibernateUtil.beginTransaction();
Query q1 = session.createQuery("from patient");
allpatients = q1.list();
for (int i = 0; i < allpatients.size(); i++) {
patient pt = (patient) allpatients.get(i);
System.out.println(pt.getLastName());
}
HibernateUtil.CommitTransaction();
}
}
Step : 5
Please remember from the above step, it will retrieve all the columns from the DB, you can check this by printing each value. But suppose if we want to select Firstname, lastname, then how we can do that ? Let us try now
package mypack;
import java.util.List;
import org.hibernate.Query;
import org.hibernate.Session;
import Utility.HibernateUtil;
public class Test {
public static void main(String[] args) {
getAllRecords();
}
public static void getAllRecords() {
List<patient> allpatients = null;
Session session = HibernateUtil.beginTransaction();
Query q1 = session.createQuery("Select firstName, lastName from patient");
for (int i = 0; i < allpatients.size(); i++) {
patient pt = (patient) allpatients.get(i);
System.out.println(pt.getLastName());
}
allpatients = q1.list();
HibernateUtil.CommitTransaction();
}
}If we now run this, then it will throw error, just let us remove the for loop and see
package mypack;
import java.util.List;
import org.hibernate.Query;
import org.hibernate.Session;
import Utility.HibernateUtil;
public class Test {
public static void main(String[] args) {
getAllRecords();
}
public static void getAllRecords() {
List<patient> allpatients = null;
Session session = HibernateUtil.beginTransaction();
Query q1 = session.createQuery("Select firstName, lastName from patient");
allpatients = q1.list();
HibernateUtil.CommitTransaction();
}
}Now it will compile and run even though there is no output.
So what is happening ? Here is the concept
if you use select, then it becomes Native SQL Query. So the output will not be your collection of patient objects, instead of that it will return list of object arrays. So we need to change our for loop as follows
package mypack;
import java.util.Iterator;
import java.util.List;
import org.hibernate.Query;
import org.hibernate.Session;
import Utility.HibernateUtil;
public class Test {
public static void main(String[] args) {
getAllRecords();
}
public static void getAllRecords() {
List allpatients;
Session session = HibernateUtil.beginTransaction();
Query q1 = session.createQuery("Select firstName, lastName from patient");
allpatients= q1.list();
for (Iterator it = allpatients.iterator(); it.hasNext(); ) {
Object[] myResult = (Object[]) it.next();
String firstName = (String) myResult[0];
String lastName = (String) myResult[1];
System.out.println( "Found " + firstName + " " + lastName );
}
HibernateUtil.CommitTransaction();
}
}Now if you run , you can see the output as follows
Hibernate: select patient0_.firstname as col_0_0_, patient0_.lastname as col_1_0_ from patient patient0_
Found Simth John
Found ROBERT DAVID
Found RICHARD JOSEPH
Found Mark Anderson
Step : 6
What is the alternate way to retrieve as patient objects ?
Here you go
Query q1 = session.createQuery("Select new patient(firstName,lastName) from patient");
Before this step, we should have proper constructor to create object with only two values. Now let us go back to our patient.java and add the constructor as follows
package mypack;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.Table;
import javax.persistence.Column;
import javax.persistence.Transient;
@Entity
@Table(name = "patient")
public class patient {
private Integer id;
private String firstName;
private String lastName;
private String password;
private String email;
private String address1;
private String address2;
private String city;
private String state;
private String zipCode;
//Our default Constructor
public patient()
{
}
//constructor with only two columns
public patient(String fname, String lname)
{
this.firstName= fname;
this.lastName = lname;
}
@Transient
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
@Id
@GeneratedValue
@Column(name = "ID")
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
@Column(name = "firstname")
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
@Column(name = "lastname")
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getAddress1() {
return address1;
}
public void setAddress1(String address1) {
this.address1 = address1;
}
public String getAddress2() {
return address2;
}
public void setAddress2(String address2) {
this.address2 = address2;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
public String getZipCode() {
return zipCode;
}
public void setZipCode(String zipCode) {
this.zipCode = zipCode;
}
}
Step : 7
Let us modify our test.java file as follows
package mypack;
import java.util.Iterator;
import java.util.List;
import org.hibernate.Query;
import org.hibernate.Session;
import Utility.HibernateUtil;
public class Test {
public static void main(String[] args) {
getAllRecords();
}
public static void getAllRecords() {
List allpatients;
Session session = HibernateUtil.beginTransaction();
Query q1 = session.createQuery("Select new patient(firstName,lastName) from patient");
allpatients = q1.list();
for (int i = 0; i < allpatients.size(); i++) {
patient pt = (patient) allpatients.get(i);
System.out.println(pt.getLastName());
System.out.println(pt.getFirstName());
}
HibernateUtil.CommitTransaction();
}
}Now you can run and see the output. Now it retrieves as patient objects
ZK MVVM CRUD - Part 1
Step 1 :
Set up the ZK + Hibernate setup as explained in this post.
Step 2 :
Initial Project structure
Step 3:
Create Mysql Table as follows
CREATE TABLE `practice` ( `Practice_ID` BIGINT(20) NOT NULL AUTO_INCREMENT, `PRACTICE_NAME` VARCHAR(255) DEFAULT NULL, `NPI_NUMBER` VARCHAR(255) DEFAULT NULL, `EMAIL` VARCHAR(255) DEFAULT NULL, `ADDRESS1` VARCHAR(255) DEFAULT NULL, `ADDRESS2` VARCHAR(255) DEFAULT NULL, `CITY` VARCHAR(255) DEFAULT NULL, `COUNTRY_GEO` VARCHAR(255) DEFAULT NULL, `POSTAL_CODE` VARCHAR(255) DEFAULT NULL, `POSTAL_CODE_EXT` VARCHAR(255) DEFAULT NULL, `STATE_PROVINCE_GEO` VARCHAR(255) DEFAULT NULL, `FAX_NUMBER` VARCHAR(255) DEFAULT NULL, `OFFICE_EXT` VARCHAR(255) DEFAULT NULL, `OFFICE_PHONE` VARCHAR(255) DEFAULT NULL, `PracticeLoginURL` VARCHAR(25) NOT NULL, `IS_ACTIVE` BIT(1) DEFAULT NULL, `CREATED_BY` BIGINT(20) DEFAULT NULL, `CREATE_TX_TIMESTAMP` DATETIME NOT NULL, `UPDATE_BY` BIGINT(20) DEFAULT NULL, `UPDATED_TX_TIMESTAMP` DATETIME DEFAULT NULL, PRIMARY KEY (`Practice_ID`), UNIQUE KEY `UniqueLoginURL` (`PracticeLoginURL`) ) ENGINE=INNODB DEFAULT CHARSET=utf8
Insert some records as follows
INSERT INTO practice(practice_name,city,POSTAL_CODE,STATE_PROVINCE_GEO) VALUES('eCosmos','City1','1111','St1') INSERT INTO practice(practice_name,city,POSTAL_CODE,STATE_PROVINCE_GEO,PracticeLoginURL) VALUES('bPractice2','City2','2222','St2','url1') INSERT INTO practice(practice_name,city,POSTAL_CODE,STATE_PROVINCE_GEO,PracticeLoginURL) VALUES('cPractice2','City2','2222','St2','url2') INSERT INTO practice(practice_name,city,POSTAL_CODE,STATE_PROVINCE_GEO,PracticeLoginURL) VALUES('dPractice2','City2','2222','St2','url3')
Step 4:
Prepare the View Part in MVVM . i.e create the ZUL File as follows
Here is the source code for practiceList.zul file
<?page title="Practice List" contentType="text/html;charset=UTF-8"?> <zk> <window title="Practice List" border="normal"> <div> <button label="Add Practice" /> </div> <separator /> <groupbox height="40px"> <label value="Practice Name" /> <space /> <space /> <textbox id="practicefilter" cols="50" /> <button id="gobutton" label="Go" /> <space spacing="20px" /> <checkbox id="activechk" label="Show only active" checked="true" /> <space spacing="20px" /> </groupbox> <separator /> <listbox id="PracticeList"> <listhead sizable="true"> <listheader label="Practice Name" sort="auto" /> <listheader label="City" sort="auto" /> <listheader label="State" sort="auto" /> <listheader label="Zip Code" sort="auto" /> </listhead> </listbox> </window> </zk>
Remember, we did not put any binding concept at this point , we will do it later stage. Now just run this zul file and check whether you get the following output

Step 5:
Now let us create Java Bean Pojo for Practice as shown
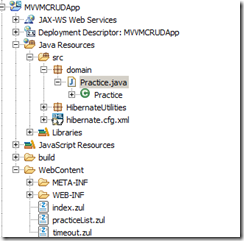
Here is the Code
package domain; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.Id; import javax.persistence.Table; @Entity @Table(name = "practice") public class Practice { private Integer practiceID; private String practiceName; private String city; private String ZipCode; private String state; private Integer isActive; @Id @GeneratedValue @Column(name = "PRACTICE_ID") public Integer getPracticeID() { return practiceID; } public void setPracticeID(Integer practiceID) { this.practiceID = practiceID; } @Column(name = "PRACTICE_NAME") public String getPracticeName() { return practiceName; } public void setPracticeName(String practiceName) { this.practiceName = practiceName; } @Column(name = "CITY") public String getCity() { return city; } public void setCity(String city) { this.city = city; } @Column(name = "POSTAL_CODE") public String getZipCode() { return ZipCode; } public void setZipCode(String zipCode) { ZipCode = zipCode; } @Column(name = "STATE_PROVINCE_GEO") public String getState() { return state; } public void setState(String state) { this.state = state; } @Column(name = "IS_ACTIVE") public Integer getIsActive() { if (isActive== null) return 1; else return isActive; } public void setIsActive(Integer isActive) { this.isActive = isActive; } }
<mapping class="domain.Practice" />
Step 6:
Now let us create our DAO Class. Please remember still we didn't touch the MVVM + Data binding. Just we are going reverse and keep every thing is ready before we connect our VIEW with MVVM Data binding
Let us create our practiceDAO Class as shown
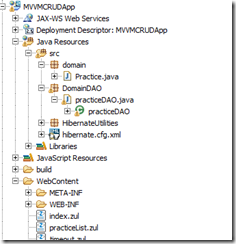
package DomainDAO; import java.util.List; import org.hibernate.Query; import org.hibernate.Session; import domain.Practice; import HibernateUtilities.HibernateUtil; public class practiceDAO { public List<Practice> getListingItems() { List<Practice> practiceListing = null; try { Session session = HibernateUtil.beginTransaction(); Query q1 = session.createQuery("from Practice "); practiceListing = q1.list(); HibernateUtil.CommitTransaction(); } catch (RuntimeException e) { if (HibernateUtil.getSession().getTransaction() != null) { HibernateUtil.rollbackTransaction(); } e.printStackTrace(); } return practiceListing; } }
Step 7:
Now let us move to MVVM. Now we will create ViewModel as shown here.
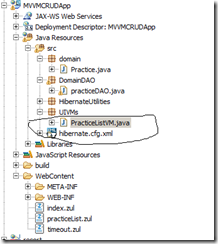
Here is the code PracticeListVM
package UIVMs; import domain.Practice; import java.util.List; import DomainDAO.practiceDAO; public class PracticeListVM { private List<Practice> AllPracticeInDB = null; public List<Practice> getprlist() { AllPracticeInDB= new practiceDAO().getListingItems(); return AllPracticeInDB; } }
Step 8:
Now let us modify our view practiceList.zul to take care of MVVM + New data binding
<?page title="Practice List" contentType="text/html;charset=UTF-8"?> <zk> <window apply="org.zkoss.bind.BindComposer" viewModel="@id('vm') @init('UIVMs.PracticeListVM')"> <div> <button label="Add Practice" /> </div> <separator /> <groupbox height="40px"> <label value="Practice Name" /> <space /> <space /> <textbox id="practicefilter" cols="50" /> <button id="gobutton" label="Go" /> <space spacing="20px" /> <checkbox id="activechk" label="Show only active" checked="true" /> <space spacing="20px" /> </groupbox> <separator /> <listbox id="PracticeList" model="@load(vm.prlist)"> <listhead sizable="true"> <listheader label="Practice Name" sort="auto" /> <listheader label="City" sort="auto" /> <listheader label="State" sort="auto" /> <listheader label="Zip Code" sort="auto" /> </listhead> <template name="model" var="p1"> <listitem> <listcell label="@load(p1.practiceName)" /> <listcell label="@load(p1.city)" /> <listcell label="@load(p1.state)" /> <listcell label="@load(p1.zipCode)" /> </listitem> </template> </listbox> </window> </zk>
Step 9:
Now run our practiceList.zul file , you should get the following output

Next Part 2
Java List and ArrayList
What is Difference between List and ArrayList
For the handling of objects collection in Java, Collection interface have been provided.
This is avail in java.util package.
"List" is an interface, extends collection interface, provides some sort of extra methods
than collection interface to work with collections. Where as "ArrayList" is the actual
implementation of "List" interface.
So both the following are correct
List x= new ArrayList(); or ArrayList x= new ArrayList();
List:
List is an interface in collection framework. several classes like ArrayList,
LinkedList implement this interface. List is an ordered collection ,
so the position of an object does matter.
ArrayList:
An ArrayList is a class which can grow at runtime. you can store java objects in an ArrayList
and also add new objects at run time.
you will use ArrayList when you dont have to add or remove objects frequently from it.
Because when you remove an object, all other objects need to be repositioned inside the ArrayList,
if you have this kind of situation, try using LinkedList instaed.
Example:
import java.util.Arrays; import java.util.Iterator; import java.util.List; public class ArrayToList { public static void main(String[] argv) { String sArray[] = new String[] { "Array 1", "Array 2", "Array 3" }; // convert array to list List<String> lList = Arrays.asList(sArray); // iterator loop System.out.println("#1 iterator"); Iterator<String> iterator = lList.iterator(); while (iterator.hasNext()) { System.out.println(iterator.next()); } // for loop System.out.println("#2 for"); for (int i = 0; i < lList.size(); i++) { System.out.println(lList.get(i)); } // for loop advance System.out.println("#3 for advance"); for (String temp : lList) { System.out.println(temp); } // while loop System.out.println("#4 while"); int j = 0; while (j < lList.size()) { System.out.println(lList.get(j)); j++; } } }
import java.util.Iterator; import java.util.List; import java.util.ArrayList; public class ListExample { public static void main(String[] args) { // List Example implement with ArrayList List<String> ls=new ArrayList<String>(); ls.add("one"); ls.add("Three"); ls.add("two"); ls.add("four"); Iterator it=ls.iterator(); while(it.hasNext()) { String value=(String)it.next(); System.out.println("Value :"+value); } } }
Annotate non persistent properties with the @Transient Annotation
In Most of the cases, all the Java bean properties (example, firstname, lastname, email, etc.) that need to be stored to the database. But in some situation, we might have other properties that do not need to be stored in the database. In this case, we need to say to hibernate that do not take this property for all the Database operation. This can be done using @Transient annotation.
By default, all fields in your class will be persisted to the database if possible. If there is a field that should not be recorded in the database, mark that field with the @Transient annotation
Now let us do an example on the above subject
Step 1:
Create the hibernate setup as shown here;
Step 2:
Now let us modify our patient.java by adding a non persistent field
package mypack;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.Table;
import javax.persistence.Column;
import javax.persistence.Transient;@Entity
@Table(name = "patient")
public class patient {private Integer id;
private String firstName;
private String lastName;
private String password;@Transient
public String getPassword() {
return password;
}public void setPassword(String password) {
this.password = password;
}@Id
@GeneratedValue
@Column(name = "ID")
public Integer getId() {
return id;
}public void setId(Integer id) {
this.id = id;
}@Column(name = "firstname")
public String getFirstName() {
return firstName;
}public void setFirstName(String firstName) {
this.firstName = firstName;
}@Column(name = "lastname")
public String getLastName() {
return lastName;
}public void setLastName(String lastName) {
this.lastName = lastName;
}
}
Step 3:
Let us modify test.java to create new patient as follows
package mypack;
import java.util.List;
import org.hibernate.Query;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.Transaction;
import org.hibernate.cfg.Configuration;
import org.hibernate.service.ServiceRegistry;
import org.hibernate.service.ServiceRegistryBuilder;
import org.hibernate.HibernateException;
import Utility.HibernateUtil;
public class Test {
private static SessionFactory factory;
private static ServiceRegistry serviceRegistry;
public static void main(String[] args) {
createpatient();
getAllRecords();
}
public static void getAllRecords() {
List allpatients;
Session session = HibernateUtil.beginTransaction();
Query q1 = session.createQuery("from patient");
allpatients = q1.list();
for (int i = 0; i < allpatients.size(); i++) {
patient pt = (patient) allpatients.get(i);
System.out.println(pt.getLastName());
}
HibernateUtil.CommitTransaction();
}
public static void createpatient() {
Session session = HibernateUtil.beginTransaction();
patient p1 = new patient();
p1.setFirstName("Mark");
p1.setLastName("Anderson");
p1.setPassword("1234");
session.save(p1);
HibernateUtil.CommitTransaction();
}
}
Step 4:
Run the test.java as Java Application and the following is the output
Hibernate: insert into patient (firstname, lastname) values (?, ?)
Hibernate: select patient0_.ID as ID0_, patient0_.firstname as firstname0_, patient0_.lastname as lastname0_ from patient patient0_
John
DAVID
JOSEPH
Anderson
Another good example
public enum Gender { MALE, FEMALE, UNKNOWN }
@Entity
public Person {
private Gender g;
private long id;
@Id
@GeneratedValue(strategy=GenerationType.AUTO)
public long getId() { return id; }
public void setId(long id) { this.id = id; }
public Gender getGender() { return g; }
public void setGender(Gender g) { this.g = g; }
@Transient
public boolean isMale() {
return Gender.MALE.equals(g);
}
@Transient
public boolean isFemale() {
return Gender.FEMALE.equals(g);
}
}